1. Project:Introduction to MATLAB#
1.1. Introduction#
In this project, you will be familiar with MATLAB. Each of the computers in the HELC has Matlab installed, so you can go there to work on projects. To get started with this project, you will need to download the starter code and unzip its contents to the directory where you wish to complete the project.
Files included in this project
project1.m - MATLAB script that steps you through the project.
checkMyAnswer.m - Script that checks your code.
checkMyAnswer.mat - Data set.
data.mat - Data set.
* f.m - Functions in MATLAB.
* matrix.m - Matrix operations in MATLAB.
* piecef.m - Use if statement to give a piecewise function.
* sumUp.m - Use for loop to calculate a Sigma sum.
* ftl.m - Use while loop to calculate a factorial.
You need to complete the files with *.
Throughout the project, you will be using the script project1.m
. This script set up all dataset for the problem and make calls to functions that you will write. Do not modify it. You are only required to modify functions in * files, following the instructions in this project.
Where to get help
At the MATLAB command line, typing help
followed by a function name displays documentation for a built-in function. For example, help plot
will bring up help information for plotting.
1.2. Assignments#
Part 1 - Function in MATLAB#
The first part of project1.m
gives you practice with MATLAB syntax. In the file f.m
, you will find the outline of a MATLAB function. Modify it to return the function
Hint
This function should work for both scalar \(x\) and vector \(\mathbf{x}\). Check your code and use the proper element-by-element operators.
Use the built-in
exp()
to calculate the natural exponential function.Use the built-in
sin()
to calculate the sine function.Use semicolon
;
to suppress the output.
Solution
y = 6 - 4*x.^2 + 3./(x+2) - exp(2*x) + sin(x.^2);
Once complete, run project1.m
(assuming you are in the correct directory, type project1
in the command window of MATLAB). You should see output similar to the following:
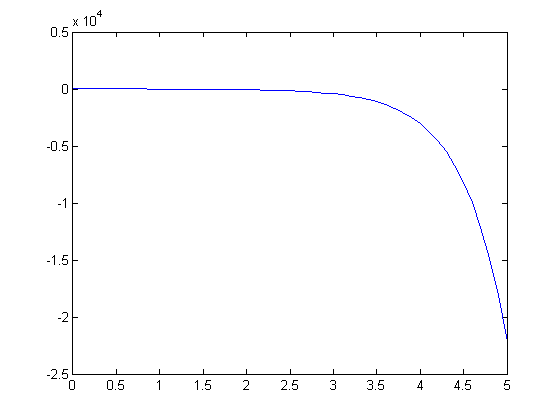
Part 2 - Matrix operations in MATLAB#
In this part of project, you will implement matrix operations to evaluate a matrix function. Modify the file matrix.m
to return a matrix \(A\) as follows
Hint
Matlab is case sensitive.
These are matrix arithmetic operations. Do not use element-by-element operators.
Use single quote
'
to calculate the transpose of a matrix.
Solution
A = 2*B - C*D';
Once complete, run project1.m
. You should see output
A=
-34 -32 0
-12 -18 -4
-68 -67 -25
Part 3 - Use if
statement for a piecewise function#
In this part, you will use if
statement to express a piecewise function. Modify the file piecef.m
to return the following function
Hint
When you write the code for the second piece, note that MATLAB does not accept the expression like
0<=x<=2
, it should bex>=0 && x<=2
. However, if the code runs into the second piece, it means \(x\) is not less than 0, sox>=0
is automatically true. In a nutshell, the condition expression for the seconde piece is justx<=2
.When you write the code for the last piece, i.e. the
else
piece, note that there is no condition expression behindelse
.
Solution
if x < 0
y = x + 1;
elseif x <= 2
y = x.^2 - 3*x + 1;
else
y = -2./x;
end
Once complete, run project1.m
. You should see output similar to the following:
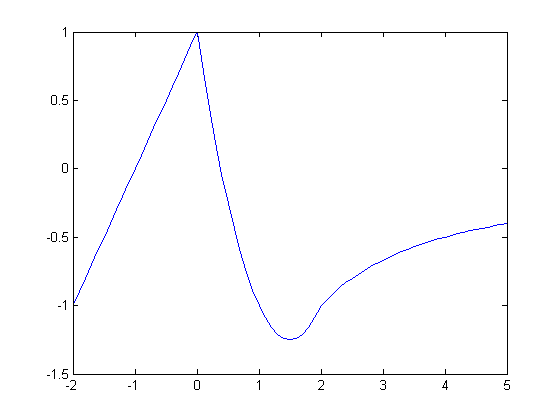
Part 4 - Use for
loop to calculate a Sigma sum#
In this part, you will use for
loop to express a sum. Modify the file sumUp.m
to return the following sum
Hint
Use
x(i)
to get the i-th component of the vectorx
.Use the built-in function
length()
to obtain the length of a vector.
Solution
for i = 1:length(x)
s = s + x(i);
end
Once complete, run project1.m
. You should see output like:
1+2+...+9+10 = 55
See also
This is a simple practice to use for
loop. MATLAB does have a built-in sum
function to calculate the sum of array elements. Check out here
Part 5 - Use while
loop to calculate a factorial#
In this part, you will use while
loop to evaluate a factorial. Modify the file ftl.m
to return \(n!\), the factorial of \(n\).
Hint
You can reduce
n
in each loop until it gets 1. So the condition expression can be set aswhile n>0
.Note that the initial value of
y
is 1 while in last section the initial value ofs
is 0.
Solution
while n > 0
y = y*n;
n = n - 1;
end
Once complete, run project1.m
. You should see output like:
10! =3628800
1.3. Submit your code#
This is the last step. You should submit your code files to WTClass. After clicking Browse My Computer, you should select all files listed in Introduction to upload. Failure to do so will affect your grade.
Click Browse My Computer:
Select all files listed in Introduction to upload